Mẹo When they have the same name, variables within ____ of a class override the classs fields
Thủ Thuật về When they have the same name, variables within ____ of a class override the classs fields Mới Nhất
Lê My đang tìm kiếm từ khóa When they have the same name, variables within ____ of a class override the classs fields được Cập Nhật vào lúc : 2022-10-25 23:36:07 . Với phương châm chia sẻ Thủ Thuật Hướng dẫn trong nội dung bài viết một cách Chi Tiết 2022. Nếu sau khi Read Post vẫn ko hiểu thì hoàn toàn có thể lại phản hồi ở cuối bài để Admin lý giải và hướng dẫn lại nha.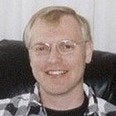
- Learn how to to initialize Java classes and objects for successful JVM executionHow to initialize a Java classReferencing class fieldsClass initialization blocksCombining class field initializers and class initialization blocks
About |
A beginner's library for learning about essential Java programming concepts, syntax, APIs, and packages.
Learn how to to initialize Java classes and objects for successful JVM execution
Classes and objects in Java must be initialized before they are used. You've previously learned that class fields are initialized to default values when classes are loaded and that objects are initialized via constructors, but there is more to initialization. This article introduces all of Java's features for initializing classes and objects.
tải về
Download the source code for example applications in this tutorial. Created by Jeff Friesen for JavaWorld.
How to initialize a Java class
Before we explore Java's support for class initialization, let's recap the steps of initializing a Java class. Consider Listing 1.
Listing 1. Initializing class fields to default valuesclass SomeClass static boolean b; static byte by; static char c; static double d; static float f; static int i; static long l; static short s; static String st;Listing 1 declares class SomeClass. This class declares nine fields of types boolean, byte, char, double, float, int, long, short, and String. When SomeClass is loaded, each field's bits are set to zero, which you interpret as follows:
false 0 u0000 0.0 0.0 0 0 0 nullThe previous class fields were implicitly initialized to zero. However, you can also explicitly initialize class fields by directly assigning values to them, as shown in Listing 2.
Listing 2. Initializing class fields to explicit valuesclass SomeClass static boolean b = true; static byte by = 1; static char c="A"; static double d = 2.0; static float f = 3.0f; static int i = 4; static long l = 5000000000L; static short s = 20000; static String st = "abc";Each assignment's value must be type-compatible with the class field's type. Each variable stores the value directly, with the exception of st. Variable st stores a reference to a String object that contains abc.
Referencing class fields
When initializing a class field, it's legal to initialize it to the value of a previously initialized class field. For example, Listing 3 initializes y to x's value. Both fields are initialized to 2.
Listing 3. Referencing a previously declared fieldclass SomeClass static int x = 2; static int y = x; public static void main(String[] args) System.out.println(x); System.out.println(y);However, the reverse is not legal: you cannot initialize a class field to the value of a subsequently declared class field. The Java compiler outputs illegal forward reference when it encounters this scenario. Consider Listing 4.
Listing 4. Attempting to reference a subsequently declared fieldclass SomeClass static int x = y; static int y = 2; public static void main(String[] args) System.out.println(x); System.out.println(y);The compiler will report illegal forward reference when it encounters static int x = y;. This is because source code is compiled from the top down, and the compiler hasn't yet seen y. (It would also output this message if y wasn't explicitly initialized.)
Class initialization blocks
In some cases you may want to perform complex class-based initializations. You will do this after a class has been loaded and before any objects are created from that class (assuming that the class isn't a utility class). You can use a class initialization block for this task.
A class initialization block is a block of statements preceded by the static keyword that's introduced into the class's body toàn thân. When the class loads, these statements are executed. Consider Listing 5.
Listing 5. Initializing arrays of sine and cosine valuesclass Graphics static double[] sines, cosines; static sines = new double[360]; cosines = new double[360]; for (int i = 0; i < sines.length; i++) sines[i] = Math.sin(Math.toRadians(i)); cosines[i] = Math.cos(Math.toRadians(i));Listing 5 declares a Graphics class that declares sines and cosines array variables. It also declares a class initialization block that creates 360-element arrays whose references are assigned to sines and cosines. It then uses a for statement to initialize these array elements to the appropriate sine and cosine values, by calling the Math class's sin() and cos() methods. (Math is part of Java's standard class library. I'll discuss this class and these methods in a future article.)
Combining class field initializers and class initialization blocks
You can combine multiple class field initializers and class initialization blocks in an application. Listing 6 provides an example.
Listing 6. Performing class initialization in top-down orderclass MCFICIB static int x = 10; static double temp = 98.6; static System.out.println("x = " + x); temp = (temp - 32) * 5.0/9.0; // convert to Celsius System.out.println("temp = " + temp); static int y = x + 5; static System.out.println("y = " + y); public static void main(String[] args)Listing 6 declares and initializes a pair of class fields (x and y), and declares a pair of static initializers. Compile this listing as shown:
javac MCFICIB.javaThen run the resulting application:
java MCFICIBYou should observe the following output:
x = 10 temp = 37.0 y = 15This output reveals that class initialization is performed in top-down order.
() methods
When compiling class initializers and class initialization blocks, the Java compiler stores the compiled bytecode (in top-down order) in a special method named
After loading a class, the JVM calls this method before calling main() (when main() is present).
Let's take a look inside MCFICIB.class. The following partial disassembly reveals the stored information for the x, temp, and y fields:
Field #1 00000290 Access Flags ACC_STATIC 00000292 Name x 00000294 Descriptor I 00000296 Attributes Count 0 Field #2 00000298 Access Flags ACC_STATIC 0000029a Name temp 0000029c Descriptor D 0000029e Attributes Count 0 Field #3 000002a0 Access Flags ACC_STATIC 000002a2 Name y 000002a4 Descriptor I 000002a6 Attributes Count 0The Descriptor line identifies the JVM's type descriptor for the field. The type is represented by a single letter: I for int and D for double.
The following partial disassembly reveals the bytecode instruction sequence for the
The instruction sequence from offset 0 through offset 2 is equivalent to the following class field initializer:
static int x = 10;The instruction sequence from offset 5 through offset 8 is equivalent to the following class field initializer:
static double temp = 98.6;The instruction sequence from offset 11 through offset 80 is equivalent to the following class initialization block:
static System.out.println("x = " + x); temp = (temp - 32) * 5.0/9.0; // convert to Celsius System.out.println("temp = " + temp);The instruction sequence from offset 83 through offset 88 is equivalent to the following class field initializer:
static int y = x + 5;The instruction sequence from offset 91 through offset 115 is equivalent to the following class initialization block:
static System.out.println("y = " + y);Finally, the return instruction offset 118 returns execution from
How to initialize objects
After a class has been loaded and initialized, you'll often want to create objects from the class. As you learned in my recent introduction to programming with classes and objects, you initialize an object via the code that you place in a class's constructor. Consider Listing 7.
Listing 7. Using the constructor to initialize an objectclass City private String name; int population; City(String name, int population) this.name = name; this.population = population; @Override public String toString() return name + ": " + population; public static void main(String[] args) City newYork = new City("Tp New York", 8491079); System.out.println(newYork); // Output: Tp New York: 8491079Listing 7 declares a City class with name and population fields. When a City object is created, the City(String name, int population) constructor is called to initialize these fields to the called constructor's arguments. (I've also overridden Object's public String toString() method to conveniently return the city name and population value as a string. System.out.println() ultimately calls this method to return the object's string representation, which it outputs.)
Before the constructor is called, what values do name and population contain? You can find out by inserting System.out.println(this.name); System.out.println(this.population); the start of the constructor. After compiling the source code (javac City.java) and running the application (java City), you would observe null for name and 0 for population. The new operator zeroes an object's object (instance) fields before executing a constructor.
As with class fields, you can explicitly initialize object fields. For example, you could specify String name = "Tp New York"; or int population = 8491079;. However, there's usually nothing to gain by doing this, because these fields will be initialized in the constructor. The only benefit that I can think of is to assign a default value to an object field; this value is used when you call a constructor that doesn't initialize the field:
int numDoors = 4; // default value assigned to numDoors Car(String make, String model, int year) this(make, model, year, numDoors); Car(String make, String model, int year, int numDoors) this.make = make; this.model = model; this.year = year; this.numDoors = numDoors;
Post a Comment